Competitive Programming (Python)
কোর্সের মেয়াদ
২ মাস
ক্লাস টাইম
১.৫ ঘণ্টা
সাপ্তাহিক ক্লাস
৩ দিন
কোর্স ফিঃ৳ ১২৯৯
মোট ক্লাসঃ২৪ টি
ক্লাস ভিডিওঃথাকবে
ভাষাঃবাংলা & English
মোট সময়ঃ৩৬ ঘণ্টা
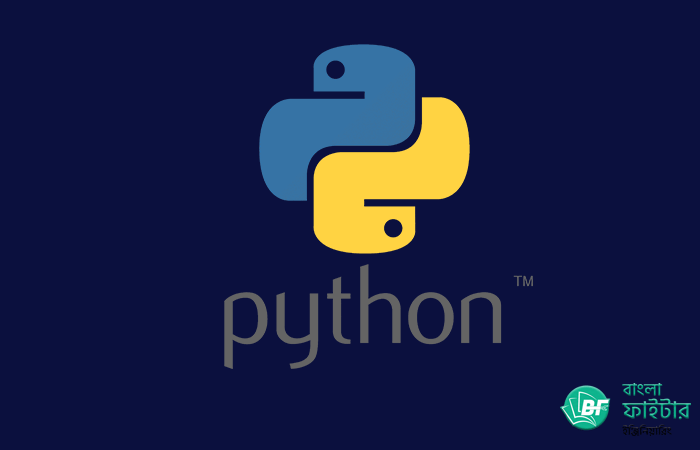
কোর্স ওভারভিউ
overview of the course
কোর্স সিলেবাস
Introduction
- Understand the Course Outline
- Development Environment Preparation
Python Basic
- Python Environment Setup
- IDE Installation
- Start Basic Programming
Data Types and Operators
- Understanding Data Types and Constants
- Working with Arithmetic Expressions
- Relational, Logical and Conditional operators
- Bitwise operators and their tricky applications
Decision-Making
- The if and if-else Statement
- Nested if and if-else Statement
- Ternary Operator
Loops
- For Loop
- While Loop
- Break, Continue
Arrays
- Defining and Initializing Arrays
- Operations -> Find, Insert, Erase, Copy, Reverse
- Subarray, Subsequence
- Multidimensional Arrays
Strings
- Arrays of Characters
- Variable-Length Character Strings
- The None String, Escape Character
- String in Python
- Palindrome, Anagram
Working with Function
- Defining a function
- Arguments and Local Variable
- Returning function results
- Global, Automatic and Static Variables
- Call by Value, Call by Reference
Sorting
- Insertion and selection sort
- Counting sort
- STL Sort
- Bubble Sort
- Merge Sort
Binary Search
- Insight of binary search and binary property
- Binary search on arrays
- Binary search on math problems
- Bisection in geometry problems
- Complexity Analysis
Recursion
- Insight, recursive equation
- Binary search with recursion
Number Theory
- GCD, LCM, Divisibility
- Primality check and generation
- Sieve of Eratosthenes
- Basic Modular Arithmetic
- Combinatorics from HSC
Problem Solve Part 1
- Leapyear
- Largest of three numbers
- Checking for Vowel
- Swapping Two Numbers
- Program to find Sum of Digits
- Largest and Smallest Number
- Program to find Factorial of number
- Fibonacci Series Program
- Factorial
Problem Solve Part 2
- Palindrome Program
- Program to reverse a String
- Program to find Average of n Numbers
- Armstrong Number
- Checking input number for Odd or Even
- Find Largest among n Numbers
- Reverse an Array
- Largest and Smallest Element in Array
- Sort Array Elements
- Addition and Subtraction of Matrices
- Matrix Multiplication
Problem Solve Part 3
- LCM And GCD of Two Numbers
- Factorial using Recursive Function
- Area of Triangle
- Celsius to Fahrenheit
- Print first n Prime Numbers
- Simple Interest
- Result Sheet
- Program to Print Pyramids
- program to make Pascal's Triangle
- Write on a File
- Count Vowel and Consonant From a File